- Home
- >
- Web Design & Development
- >
- How to do navigation in React Native web in 2022?
“React Native for Web” makes it possible to run React Native components and APIs on the web using React DOM. It is as simple as that!! So, if you have an app and you want to build a web version of it, or if you are planning to build a website along with an app, you should definitely learn React native web.
Setting up navigation in react native web is challenging as the navigation system works quite differently in apps vs browsers.
In this article, we’ll set up the most popular react-navigation on react-native-web.
Using React navigation in react native web
React navigation is the most famous library for navigation in react–native. In this section, we’ll try to integrate react-navigation in react–native–web.
Prerequisite
For this setup, we’ll be using the expo as the platform on which we’ll be building our react–native app, which will run on android, ios, and the web.
Make sure you have expo-cli
installed it already.
To set up your codebase using expo, check this GitHub link [master branch]. Simply clone the branch and do run expo start
If you are looking for a quick code, then you can check this GitHub link [NOTE: the branch is react–navigation–setup and not master.]
Installation
Run the following command to install react–navigation along with other needed packages including react–navigation–stack.
expo install react-navigation react-native-gesture-handler react-native-reanimated react-native-screens
npm i react-navigation-stack
npm i @react-navigation/web
Check your package.json files to ensure all of the above packages are installed, make sure react–navigation is version 4+. If you are wondering, why did we use expo instead of NPM/yarn while installing react–navigation, then the reason is that expo would look for the correct version of the react–navigation libraries that’d work with the expo version that’s installed in your project.
If you look into the printed console it uses NPM underneath.

Create a few screens
Now, let’s set up a few screens to test our navigation flow:
// feed screen
import React from 'react';
import {View, Text, StyleSheet, Button, Platform} from 'react-native';
export default class Feed extends React.Component {
render() {
return <View style={styles.container}>
<Text>This is the feed screen</Text>
<Button
title="Go to Profile"
onPress={() => this.props.navigation.navigate('Profile')}
/>
</View>
}
}
const styles = StyleSheet.create({
container: {
flex: 1,
justifyContent: 'center',
alignItems: 'center',
}
})
The above is the code for FeedScreen, which is a simple text, and a button. The button when clicked should go directly to the profile screen.
// Profile screen
import React from 'react';
import {View, Text, StyleSheet, Button, Platform} from 'react-native';
export default class Profile extends React.Component {
render() {
return <View style={styles.container}>
<Text>This is the profile screen</Text>
<Button
title="Go to Feed"
onPress={() => this.props.navigation.navigate('Feed')}
/>
</View>
}
}
const styles = StyleSheet.create({
container: {
flex: 1,
justifyContent: 'center',
alignItems: 'center',
}
})
The Profile screen is the same as the Feed screen. Both the screens have a button which takes to the other screen.
Let’s also create stack navigation to connect these two screens together:
// Home.js, just a name of the stack navigation
import {createStackNavigator} from 'react-navigation-stack';
import {createAppContainer} from 'react-navigation';
import Feed from "../screens/Feed";
import Profile from "../screens/Profile";
const Home = createStackNavigator(
{
Profile: Profile,
Feed: Feed,
},
{
navigationOptions: {
headerTintColor: '#fff',
headerStyle: {
backgroundColor: '#000',
},
},
}
);
const container = createAppContainer(Home);
export default container;
Since the object that’s passed to CreateStackNavigator
Profile
comes first, the Profile screen is the default screen of this stack navigator.
Now, in the App.js file, simply render the Home
Navigation.
// App.js
export default function App() {
return (
<View style={styles.outer}>
<Home/>
</View>
);
}
Just run the app using command expo start
, and it should launch the expo bundler for you.
If you press i
to launch the Expo app in the IOS simulator in the terminal, the following screen comes up on display, if everything goes well.
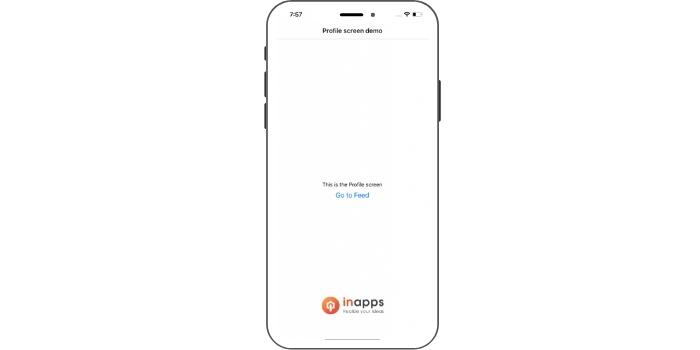
When you click on the Go to Feed
button, it should take you to the feed screen.
To run the same setup on to the web, simply press w
in your terminal, it will launch the web app in your default browser.

The click functionality also works on the web as well. The top border is for the screen title, you can add it by adding navigationOptions
to the feed and profile screen like this.
export default class Feed extends React.Component {
static navigationOptions = {
title: "Feed"
}
...
But there is one problem with the web navigation, the URL doesn’t change when you go from the profile to the feed screen.
In the web navigation, it is extremely important to have the change in page reflecting in the URL as well.
In the app, there is no way a user can directly jump to a screen other than the default screen, but in the browser it is possible, a user can enter a URL.
The good part of react–navigation is that it supports URL updates when the screen changes. The way navigationOptions
is added inside the screen class similarly you can also add title
.
export default class Feed extends React.Component {
static navigationOptions = {
title: "Feed"
}
static path = "feed";
render() {
...
For the profile screen, you can keep the path as empty static path = ""
.
When you go to http://localhost:19006/feed, the web app would understand that you want to go to the feed screen and will render that for you. Try going to http://localhost:19006/feed directly, and it should render the feed page for you.
But when you click on the Go to Feed
button, the URL won’t change.
There are a few other things that we need to do to make this work:@react-navigation/web
also provide Link
module which gets converted into a
tag on to the web.
And, this module doesn’t work when you try to run the app. So, we use Platform
module provided by react–native to differentiate between web and app.
// feed screen
import React from 'react';
import {View, Text, StyleSheet, Button, Platform} from 'react-native';
import {Link} from "@react-navigation/web";
const isWeb = Platform.OS === 'web';
export default class Feed extends React.Component {
static navigationOptions = {
title: "Feed"
}
static path = "feed";
render() {
return <View style={styles.container}>
<Text>This is the feed screen</Text>
{
!isWeb ? <Button
title="Go to Profile"
onPress={() => this.props.navigation.navigate('Profile')}
/>: <Link routeName="Profile">Go Profile</Link>
}
</View>
}
}
const styles = StyleSheet.create({
container: {
flex: 1,
justifyContent: 'center',
alignItems: 'center',
}
})
Here, we are conditionally rendering the Link and the Button.
You need to do similar changes for the Profile screen as well. Also, in the navigation container, instead of createAppContainer
you need to use createBrowserApp
for the web.
Here is the code for the navigation:
// Home.js, just a name of the stack navigation
import {createStackNavigator} from 'react-navigation-stack';
import {createAppContainer} from 'react-navigation';
import {createBrowserApp} from '@react-navigation/web';
import Feed from "../screens/Feed";
import Profile from "../screens/Profile";
import {Platform} from "react-native";
const isWeb = Platform.OS === 'web';
const Home = createStackNavigator(
{
Profile: Profile,
Feed: Feed,
},
{
navigationOptions: {
headerTintColor: '#fff',
headerStyle: {
backgroundColor: '#000',
},
},
}
);
const container = isWeb ? createBrowserApp(Home): createAppContainer(Home);
export default container;
Try running the app in the browser, Go to feed
button click should change the URL to http://localhost:19006/feed.
Also, the app should be running fine on the simulators as well.
In the following videos, I have done the walkthrough of the react-navigation setup on react native web.
Conclusion
We hope this tutorial has helped you in learning how to do navigation in React-native web. It’s not as difficult as you think it. Just go through the entire process once and practically implement it. Also, in case of any queries, you can contact InApps. We would love to help you.
FAQs
- What is the way to navigate in React native web?
You need to install it. Then, run that command to install react-navigation along with other required packages comprising react-navigation-stack. Keep checking your package .json files to make sure all the packages are installed, and ensure react-navigation is version 4+.
- Can we use React Navigation in React JS?
If you are well-versed with JavaScript, React native, and React, then you will be able to easily go ahead with React navigation swiftly.
- What is navigation in React JS?
js routing. The link part which is offered by React-router-dom assists in making anchor tags that will help you navigate without re-loading that page alone. If you use the anchor tag given by HTML, it will reload the page while navigating.
Learn more: Top 12 React Dev Tools For 2022
Let’s create the next big thing together!
Coming together is a beginning. Keeping together is progress. Working together is success.